基于 hash 算法
import os
import sys
import hashlib
def compute_hash(file_path):
with open(file_path, 'rb') as file:
content = file.read()
hash_object = hashlib.md5(content)
return hash_object.hexdigest()
def find_different_files(folder1, folder2):
for root, dirs, files in os.walk(folder1):
for file in files:
file_path1 = os.path.join(root, file)
file_path2 = os.path.join(folder2, os.path.relpath(file_path1, folder1))
if os.path.exists(file_path2):
hash1 = compute_hash(file_path1)
hash2 = compute_hash(file_path2)
if hash1 != hash2:
print("文件不同:", file)
print("路径1:", file_path1)
print("路径2:", file_path2)
print()
else:
print("文件不存在于folder2:", file)
print("路径1:", file_path1)
print()
# 遍历folder2,查找folder1不存在的文件
for root, dirs, files in os.walk(folder2):
for file in files:
file_path2 = os.path.join(root, file)
file_path1 = os.path.join(folder1, os.path.relpath(file_path2, folder2))
if not os.path.exists(file_path1):
print("文件不存在于folder1:", file)
print("路径2:", file_path2)
print()
if __name__ == "__main__":
if len(sys.argv) != 3:
print("请输入正确的文件夹路径作为参数!")
print("用法: file_diff <folder1> <folder2>")
os.system("pause")
else:
folder1 = sys.argv[1]
folder2 = sys.argv[2]
find_different_files(folder1, folder2)
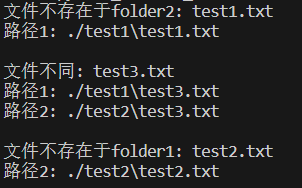
测试效果